- Published on
Getting Started in Unreal Engine 5 - Part 3
- Authors
- Name
- Hardly Brief Dan
- @HardlyBriefDan/
Introduction
In this third part of the tutorial series, we are going to add Player Health
to our character, we will create an On Death Event
to handle logic for when the character's heath reaches 0, we will add a Ragdoll Effect
for when player dies, and finally we will Reset the Level
to let the player have another attempt at the puzzle.
What will be covered:
- Adding Player Health
- Creating an On Death Event and logic
- Ragdoll Effect on Player death
- Resetting the level to retry the puzzle
This series is written as both part of my Udemy Course and to accompany the sections of the course I am releasing on my Youtube channel.
Prerequisites
In order to follow along with this tutorial, I am assuming you have installed Unreal Engine 5. If you have not, you need to download and install the engine, please visit Unreal Engine Download website to get started. Epic Games created a great video to get you going.
You should have also created the project and installed the FX Pack as we did in a previous tutorial.
Adding Health to our Player Character
Now that we have our Lightning Tornado Obstacle
that launches the player into the air we also want it to apply damage to our player to reinforce how dangerous it is and that it should be avoided. Adding health to our character is relatively simple and quick process. We will create a Integer Variable
that belongs to the BP Third Person Character
. Check out my post on (Data Types and Variables)[] to learn more.
Inside the BP Third Person Character
blueprint, create a new variable called Player Health
. This variable should be an integer data type. Click Compile and Save at the top of the blueprint window and select the Player Health
variable we just created. Now, set the default value to any number such as 100.
Subtract Player Health Blueprint Function
In order to apply damage to our player, we need to create a function that enables us to subtract damage from our health. Continuing inside the BP Third Person Character
blueprint, we are going to create a new function called Apply Damage and add 1 input of type integer that we can call Amount (this is the amount of damage being applied).
This is a great spot to use an Interface and call it Damageable. The interface could have a function called Apply Damage. We are keeping it more simple in this course so we are not going to create one.
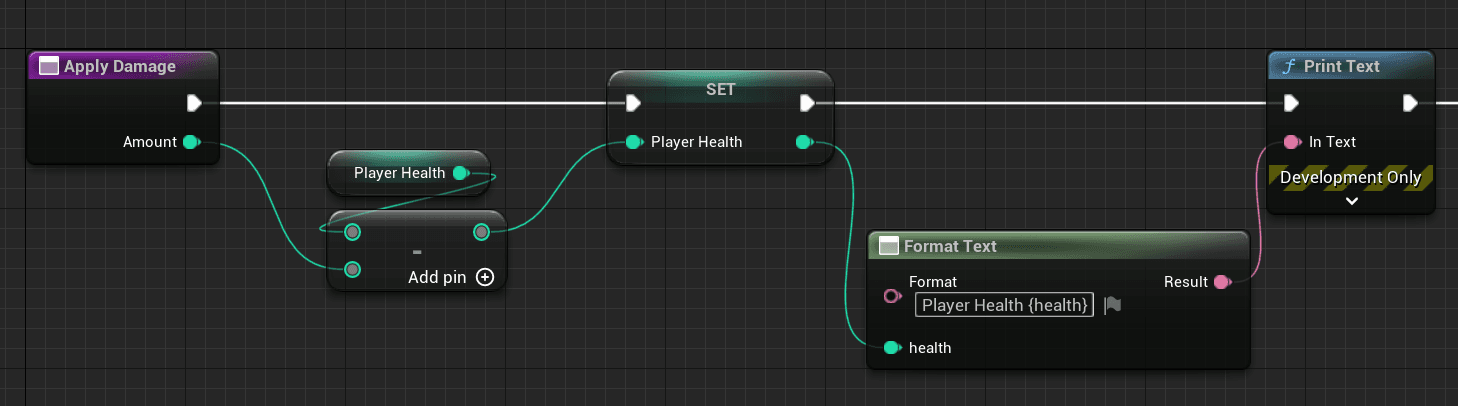
Inside the newly created function, you will want to add the above nodes to subtract the damage Amount
from the Player Health
. I also added a Format Text
node so I can output a helpful statement to the screen while playing the game to get an update on my player's health every time damage is applied to the player.
Adding a Macro to Check Player Health (Is Player Dead)
In order to finish our Apply Damage
function, we need to create a macro and a custom event. To create a macro, you can quickly add one on the left hand side of the editor under the macro section.
These are similar to functions and are a perfect place to encapsulate reusable logic such as checking if our player has died.
Name the macro IsPlayerDead
. We will use this macro to make a quick comparison to determine if our player has died. The macro will output the result of a Less than or equal
node. Once you have added the Less than or equal
, you can just drag the red bool output to the Output
node and it will auto create a output parameter. You should rename it to IsDead
.
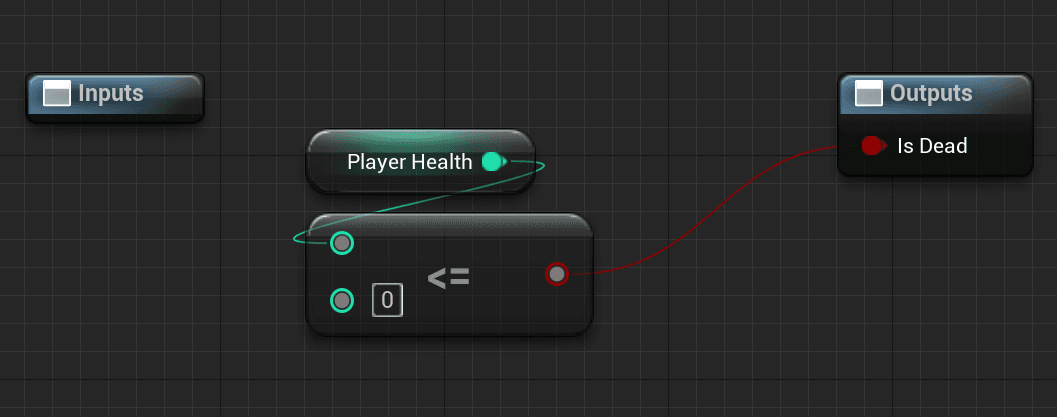
Creating an On Death Event
Now, lets add the last piece we need for our Apply Damage
function, the OnDeathEvent
. This event will be called when our player dies. Right click in the Event Graph and search for Add Custom Event. This will create a new event you can name called OnDeath
. This will create a red node. Now from here you can add the below nodes to output to the log the player has died, add a short delay for game mechanics, and lastly, set our mesh to simulate physics which will add some rag doll effects which we will discuss more on later!
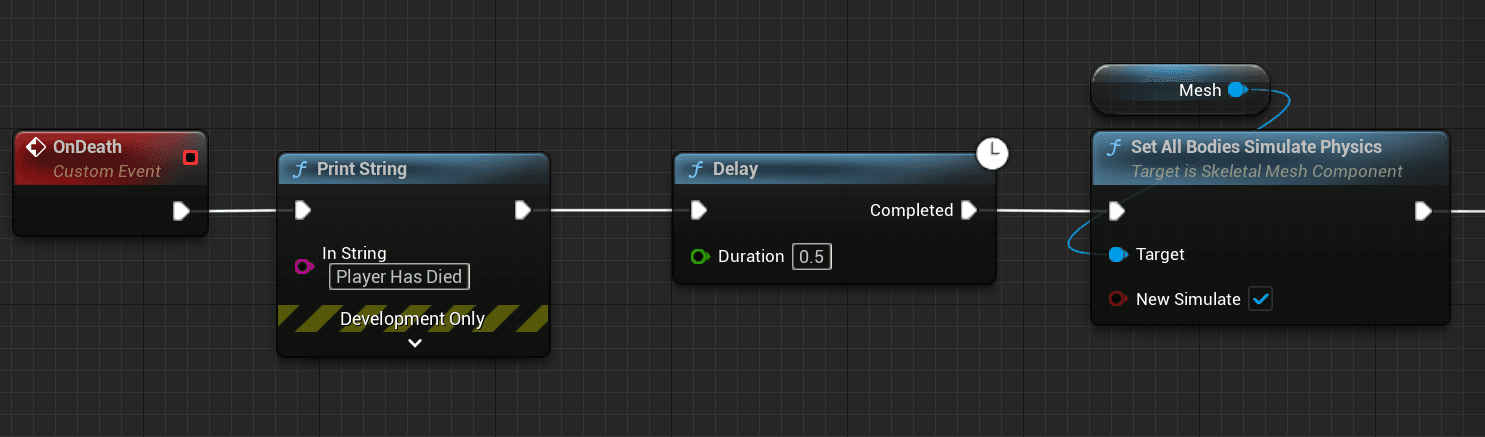
You should be able to right click in the event graph and type
Set All Bodies Simulate
and you should see an option labeled (Mesh).
Finishing our Apply Damage Function
Since we have created the IsPlayerDead
macro and added our custom event we can finish the ApplyDamage
function as shown below. You can attach the output of the macro to a Branch
node and if the result of our macro is true, our player has died, then we can call our custom event OnDeath
.
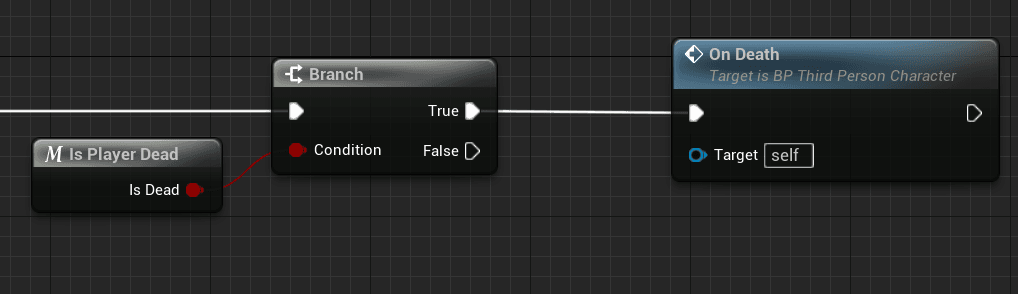
Creating a Rag Doll Effect
In order to add Rag Doll effects to our player character we need to update a setting on the player character itself. Once we update this setting, the Set All Bodies Simulate
node we created in the OnDeath
event will work properly.
- Select the Mesh (CharacterMesh0) in the Components panel on the left of the editor
- In the details panel on the right, scroll down to the Collision section
- Look for the
Collision Presets
drop down and selectRagdoll
- Compile and Save and you are good to go!
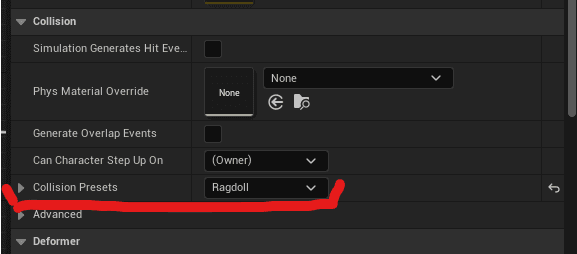
Adding a Text Widget on Player Death
Our character takes damage and can lose enough health that they die. Now we need to give the player a bit more feedback and create a simple text widget that is red and says Player Has Died
.
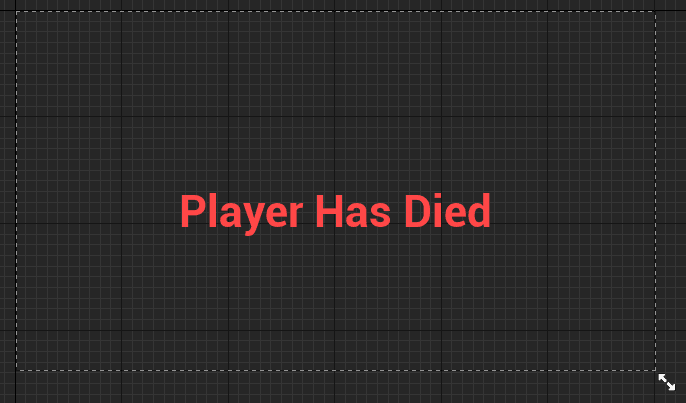
Creating widgets in Unreal Engine is extremely simple and we can do this in just a couple of steps! In the content browser do the following:
- Right click and make a new folder called
Widgets
(this is not mandatory but good to keep organized) - Double click inside the new folder
- Right click and search for Widget, select
Widget Blueprint
- From the new pop-up window, select User Widget and name is
UW_DeathText
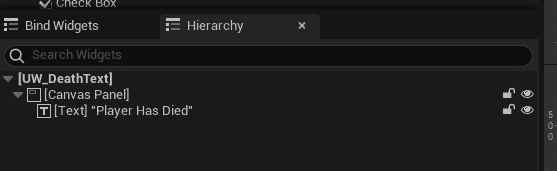
Now we can design the widget we want to create as shown above! In the search bar, type Canvas Panel
and click and drag to the Hierarchy panel. This will add a Canvas Panel
component to the widget tree. This gives you an area to place your text and is the size of the screen the game is playing on. Next, add a Text
component to the Hierarchy. This you can edit to say Player Has Died
and change the color of the red. If you select the Text
component you can also adjust alignment settings to center it within the canvas.
Resetting the Level
We have created everything we need and now we want to finish the OnDeath
event with the below nodes to do the following:
- Disable Character Movement (we don't want to move when we are dead)
- Create the
DeathText
widget and Add it to the Viewport (this displays the text on the screen) - Add a short delay to allow the player to read the text and let the rag doll effect play out
- Open Level (By Name) which is a node that will reset our level for us when the player dies

Whats Next?
We have added Player Health
to our character and used a Function and Macro to update the Health value and determine if the player has died. We created an On Death Event
to handle logic for when the player dies and inside that event we created a Ragdoll Effect
, adding a Text Widget to display on screen, and learned how to Reset the Level
.
Next up, we are going to create a Switch
so the player can deactivate the Lightning Tornado Obstacles
.
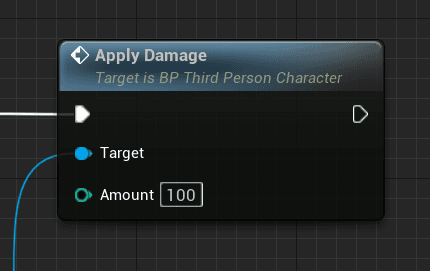
Build Your Dream Game in Unreal Engine
I am creating a course that will teach you the core systems you need to develop a Steam-ready game. The course reviews programmings fundamentals and guides you to create these foundational components using both Blueprints and C++. Be sure to Check it out today!